Building Robust Systems: A Comprehensive Guide to Embedded C Programming with 8051
Embedded c programming with 8051
Embedded systems are at the core of countless electronic devices that we rely on every day, from smartphones and innovative appliances to automotive systems and industrial machinery. At the heart of many embedded systems lies the 8051 microcontroller, a versatile and widely used platform known for its reliability and efficiency. To harness the full potential of the 8051 microcontrollers and create robust systems, mastering embedded C programming is essential.
In this comprehensive guide, we will delve into the world of embedded C programming with the 8051 microcontroller. Whether you are a beginner seeking to learn the fundamentals or an experienced programmer looking to enhance your skills, this article will provide the knowledge and insights needed to build robust and efficient embedded systems.
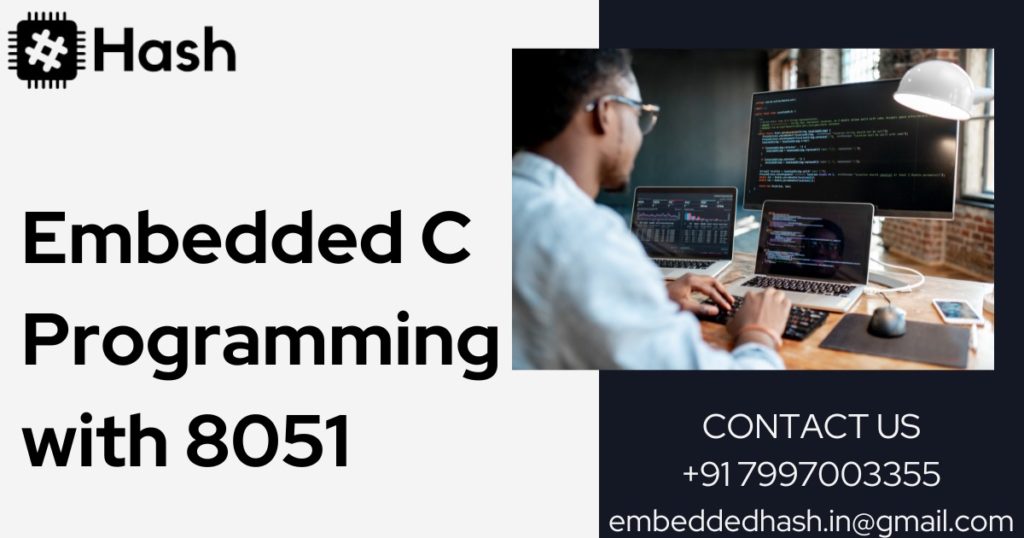
Introduction to Embedded C Programming with 8051 Microcontroller
What is Embedded C Programming?
Embedded C programming is a variant of the C programming language that is tailored for embedded systems. It provides features and libraries that allow developers to efficiently interact with the hardware and utilize the limited resources available in embedded systems. Embedded C programmers write code to perform specific tasks and control the behavior of the microcontroller.
Embedded C programming is often used in applications such as industrial automation, automotive systems, consumer electronics, and medical devices. Its key features include efficient memory management, direct register access, and support for interrupts and low-level programming.
Overview of the 8051 Microcontroller
The 8051 microcontroller is an 8-bit microcontroller family that was introduced by Intel in 1981. It has since become one of the most popular choices for embedded systems due to its simplicity, low cost, and wide availability of development tools and resources. The 8051 microcontroller is based on the Harvard architecture and features a range of peripherals such as timers, serial ports, and general-purpose I/O pins.
The 8051 microcontroller family is known for its versatility and can be found in a variety of applications, including home appliances, automotive systems, communication devices, and industrial control systems. It is highly programmable and supports a wide range of instructions and data types.
Importance of Building Robust Systems
Building robust systems is crucial in embedded programming due to the critical nature of many embedded applications. Embedded systems are often used in safety-critical environments, where a failure in the system could have serious consequences. Therefore, it is important to ensure that the software running on the microcontroller is reliable, resilient, and able to handle unexpected situations.
Robust systems are built by following best practices in software design, writing modular and maintainable code, and thoroughly testing the system for different scenarios. In embedded C programming, this involves understanding the hardware limitations, optimizing code for efficiency, and implementing error-handling mechanisms.
By building robust systems, developers can minimize the risk of system failures, improve the overall performance of the embedded system, and enhance the user experience. Additionally, robust systems are easier to maintain and troubleshoot, saving time and resources in the long run.
Getting Started with Embedded C Programming With 8051
Setting up the Development Environment
To get started with embedded C programming with the 8051 microcontroller, you’ll need to set up your development environment. Here are the steps to follow:
- Choose an Integrated Development Environment (IDE): There are several IDE options available for embedded C programming with the 8051. You can choose an IDE such as Keil µVision, SDCC (Small Device C Compiler), or IAR Embedded Workbench. These IDEs provide a user-friendly interface and tools for writing, compiling, and debugging your code.
- Install the IDE: Once you have chosen an IDE, download and install it on your computer. Follow the installation instructions provided by the IDE’s documentation. Make sure to select the appropriate options for the 8051 microcontroller.
- Install the necessary device drivers: Some IDEs may require you to install specific device drivers to communicate with the 8051 microcontroller. These drivers are usually provided by the manufacturer of the development board or programmer you are using. Follow the instructions provided by the manufacturer to install the device drivers correctly.
- Connect the development board or programmer: Connect your development board or programmer to your computer using the appropriate interface (USB, serial, etc.). Ensure that the connections are secure, and the device is powered on.
- Configure the IDE: Open the IDE and configure it for the 8051 microcontroller. Specify the target microcontroller model, clock frequency, memory size, and other relevant settings.
- Create a new project: Create a new project in the IDE and specify the name and location for your project files. This will create a workspace where you can organize your code, configuration files, and other resources.
Understanding the 8051 Architecture
Before writing your first embedded C program for the 8051 microcontrollers, it is essential to have a basic understanding of its architecture. The 8051 microcontroller is based on the Harvard architecture, which means it has separate program memory and data memory.
The key components of the 8051 architecture include:
- CPU: The central processing unit of the microcontroller, responsible for executing instructions.
- Program Memory: Stores the program instructions that the CPU fetches and executes.
- Data Memory: Used to store data and variables during program execution.
- I/O Ports: Provide interfaces to connect peripherals and external devices.
- Timers/Counters: Used for timekeeping, event counting, and generating timing signals.
- Serial Communication Ports: Enable communication with other devices using serial protocols such as UART or SPI.
Writing Your First Embedded C Program
Now that you have set up your development environment and understood the basics of the 8051 architecture, it’s time to write your first embedded C program. Here are the steps to follow:
- Open your IDE and create a new source file for your program.
- Start by including the necessary header files that provide access to the specific registers and functions of the 8051 microcontroller.
- Write your C program code. Begin with simple tasks, such as toggling an LED connected to one of the I/O ports or generating a delay using one of the timers.
- Compile your code to ensure there are no syntax errors. The IDE will check for any errors and provide error messages if there are any.
- Once your code has compiled successfully, you can flash it onto the 8051 microcontroller. This involves using the IDE and the connected development board or programmer to transfer the compiled code to the microcontroller’s program memory.
- Run your program and observe the behavior of the microcontroller. You can use debug features provided by the IDE to step through your code, set breakpoints, and monitor the register values during execution.
By following these steps, you will be able to write and run your first embedded C program for the 8051 microcontroller. As you gain more experience, you can explore more advanced features and develop more complex applications.
Essential Concepts in Embedded C Programming with 8051
Data Types and Variables
Data types are an essential concept in embedded C programming as they define the type of data that can be stored in a variable. The most commonly used data types in embedded C programming are:
- char: used to store single characters or small integers.
- int: used to store integers.
- float: used to store floating-point numbers.
- double: used to store double-precision floating-point numbers.
- void: used to represent no value or unknown data type.
Variables are used to store data values in embedded C programming. A variable is defined using a type and a unique name, which can be used to access its value. Variables are assigned values using an assignment operator (=) and can be used in expressions and control structures in the program.
Operators and Expressions
Operators and expressions are used extensively in embedded C programming. Operators are symbols or keywords that perform specific operations on operands. The most commonly used operators in embedded C programming are:
- Arithmetic Operators: used for arithmetic operations, such as addition (+), subtraction(-), multiplication (*), division (/), and modulus(%).
- Relational Operators: used to compare values, such as less than (<), greater than (>), equal to (==), not equal to (!=), less than or equal to (<=), and greater than or equal to (>=).
- Logical Operators: used to combine logical expressions, such as AND (&&), OR (||), and NOT (!).
- Bitwise Operators: used to perform operations on the individual bits of an integer value, such as AND (&), OR (|), NOT (~), XOR (^), left shift (<<), and right shift (>>).
Expressions are combinations of variables, constants, and operators that evaluate to a single value. Expressions can be used in assignments, control structures, and function calls in embedded C programming.
Control Structures (Conditional Statements and Loops)
Control structures are used to conditionally execute and repeat code in embedded C programming. The two main control structures are conditional statements and loops.
- Conditional Statements: Conditional statements allow code to be executed selectively based on a condition. The most commonly used conditional statements are if-else statements and switch statements.
- Loops: Loops allow code to be repeated a certain number of times or until a specific condition is met. The most commonly used loops are for-loops, while-loops, and do-while loops.
Control structures are used extensively in embedded C programming to implement decision-making and repetition in a program.
Functions and Modular Programming
Functions are essential in embedded C programming as they allow code to be organized into modular blocks that reduce duplication and improve readability. A function is a block of code that performs a specific task and can be called from other parts of the program.
Functions in embedded C programming are defined using a return type (if any), a function name, and a list of parameters (if any). The function body is enclosed in curly braces and contains the code that is executed when the function is called.
Modular programming is the practice of organizing code into reusable and interchangeable modules, such as functions and libraries. Modular programming improves code maintenance, scalability, and code reuse.
By mastering the above essential concepts, embedded C programmers can write efficient code and develop robust applications for the 8051 microcontroller.
Memory Management and Optimization Techniques
Memory Organization in the 8051 Microcontroller
Memory organization in the 8051 microcontroller is important to understand when it comes to managing and optimizing memory usage in embedded C programming with 8051. The 8051 microcontroller has a segmented memory model, divided into several memory spaces:
- Program Memory: Stores the program instructions that the CPU fetches and executes. In the 8051, the program memory is divided into multiple banks, each containing a fixed number of 8-bit instructions.
- Data Memory: Used to store data and variables during program execution. The data memory is further divided into three regions: Internal RAM, Special Function Register (SFR) space, and bit-addressable memory.
- External Data Memory: The 8051 microcontroller can also access external data memory through address lines and control signals. This external memory is often used for storing large amounts of data or additional program code.
Understanding the memory organization in the 8051 microcontroller allows developers to allocate and utilize memory resources efficiently.
Memory Access and Addressing Modes
Memory access and addressing modes play a crucial role in embedded C programming with 8051 microcontrollers. The 8051 microcontroller provides various addressing modes to access different types of memory.
- Direct Addressing: The simplest form of addressing, where the memory location is specified directly in the instruction.
- Register Indirect Addressing: In this mode, a register is used to hold the memory address, and the instruction operates on the data at that address.
- Indexed Addressing: Allows the use of an index register, such as the DPTR or a register pair, to indirectly access memory locations.
- Immediate Addressing: Data is included as part of the instruction itself without specifying a memory location.
- Bit Addressing: The 8051 microcontroller provides bit-addressable memory, allowing individual bits to be accessed and modified independently.
Developers need to understand and utilize the different addressing modes as per their specific requirements to optimize memory access and speed up program execution.
Optimizing Embedded C Code for Performance and Size
Optimizing embedded C code for performance and size is crucial in resource-constrained environments such as the 8051 microcontroller. Here are some techniques to consider:
- Use appropriate data types: Choose the smallest data type that can hold the required range of values to minimize memory usage.
- Minimize the use of global variables: Excessive use of global variables can consume precious memory. Instead, prefer local variables.
- Use bitwise operations: Bitwise operations can be more efficient when dealing with individual bits or flags rather than using boolean variables or multiple boolean operations.
- Avoid unnecessary conversions: Minimize unnecessary data type conversions, as they can introduce overhead and reduce performance.
- Optimize loops: Optimize loops by reducing iterations, minimizing loop overhead, and avoiding unnecessary calculations within loops.
- Use inline functions: Inline functions can eliminate the overhead of function calls and improve performance in some cases.
- Enable optimization flags: Enable compiler optimization flags to let the compiler apply various optimizations, such as loop unrolling, constant folding, or code size optimization.
- Profile and measure: Profile your code and measure its performance to identify potential bottlenecks and areas for optimization.
Dealing with Limited Resources
The 8051 microcontroller has limited resources, such as memory, I/O ports, and processing power. Here are some strategies for dealing with these limitations and optimizing resource usage:
- Optimize memory usage: Use data types and memory allocation techniques that minimize memory usage. Avoid unnecessary duplication of code or data.
- Prioritize critical functions: Identify critical functions and optimize them for performance. These functions usually include time-critical operations or frequently used functionalities.
- Use interrupts: Utilize interrupts to handle time-sensitive tasks or external events, allowing the CPU to continue with other operations.
- Use timers and counters efficiently: Take advantage of the timers and counters available in the 8051 microcontroller to offload timekeeping and event-counting tasks from the CPU.
- Optimize I/O operations: Minimize I/O operations and use efficient I/O handling techniques, such as buffering, to reduce the overhead of data transfer.
- Use code optimization techniques: Employ optimization techniques discussed earlier, such as minimizing unnecessary calculations, reducing loop iterations, and optimizing data structures.
By being mindful of limited resources and employing optimization techniques, developers can effectively utilize the capabilities of the 8051 microcontroller and develop efficient and reliable embedded C code.
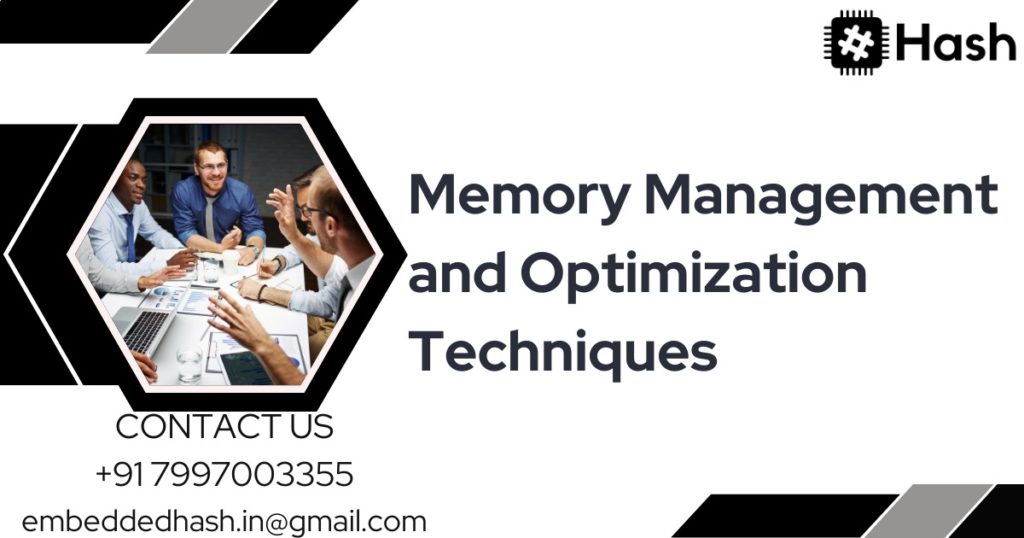
Real-World Applications and Projects
Building an LED Blinking Program
LED blinking is a common introductory project to understand the basic working of a microcontroller. In this project, you can connect an LED to one of the I/O pins of the 8051 microcontroller and write a program that toggles the LED on and off at a specific rate. This simple project helps you understand how to control digital output pins and introduce you to the process of programming and interfacing with external devices.
Creating a Digital Thermometer using a Temperature Sensor
Building a digital thermometer using a temperature sensor is another popular project that demonstrates the use of an analog sensor with the 8051 microcontroller.
You can connect a temperature sensor, such as the LM35, to one of the analog input pins of the 8051 microcontroller. Using the built-in analog-to-digital converter (ADC) of the microcontroller, you can read the analog voltage from the temperature sensor and convert it to a digital value.
By applying a simple mathematical formula, you can then convert the digital value to the temperature in Celsius or Fahrenheit and display it on an LCD screen or transmit it wirelessly using communication protocols like UART or SPI.
Developing an LCD Display Interface
Developing an LCD display interface can be a beneficial project as it allows you to integrate a user interface with the 8051 microcontroller. Many 16×2 or 20×4 LCD modules are compatible with the 8051 microcontroller and can be connected to any of the available I/O pins.
By writing a program that sends commands and data to the LCD module, you can display information such as sensor readings, messages, menu options, or any relevant output on the LCD screen. This project helps you understand the basics of interfacing with an LCD module, using the microcontroller’s I/O pins, and controlling the display using commands.
Implementing a Simple Home Automation System
Implementing a simple home automation system using the 8051 microcontroller can be an exciting and practical project. The system can include various functionalities such as controlling lights, fans, appliances, or even a security system. You can connect relay modules or solid-state relays to the I/O pins of the microcontroller to control the electrical devices.
By writing a program, you can interface with sensors like motion sensors, temperature sensors, or light sensors to automate the behavior of the connected devices. Additionally, you can include a user interface through an LCD or keypad to control and monitor the home automation system.
These real-world projects provide hands-on experience and practical applications of the 8051 microcontroller. They help you understand microcontroller programming, sensor interfacing, device control, and other aspects required to develop embedded systems. You can modify and expand upon these projects based on your specific requirements and interests to explore the capabilities of the 8051 microcontrollers further.
Advanced Topics in Embedded C Programming with 8051
Interfacing with External Memory
The 8051 microcontroller has a limited amount of internal memory, which can become insufficient for advanced projects that require large amounts of code or data storage. In such cases, external memory can be interfaced with the microcontroller to expand its memory capacity. There are several types of external memory devices, including static RAM (SRAM), dynamic RAM (DRAM), and EEPROM.
Interfacing with external memory using the 8051 microcontroller requires careful attention to the addressing scheme, data transfer protocols, and memory organization. Programs must be written to handle both internal and external memory addressing, and the microcontroller will need configuration for interfacing with specific external memory.
Real-Time Operating Systems (RTOS) for 8051
Real-time operating systems (RTOS) are becoming increasingly critical in embedded systems and the 8051 microcontroller is no exception. RTOS provides a mechanism for multitasking and scheduling tasks in real-time. It allows tasks to execute based on priorities, and the scheduling is preemptive, so higher-priority tasks can interrupt lower-priority tasks.
Interfacing the 8051 microcontroller with an RTOS requires a bit of setup, and the RTOS itself may require additional memory. Tasks and threads need to be mapped with the available memory and CPU usage. Developers must understand the RTOS, its scheduling algorithms, memory occupation issues, and how it integrates with multiple tasks to ensure stable and optimal performance.
Implementing Wireless Communication (RF, Bluetooth, etc.)
Implementing wireless communication with the 8051 microcontroller offers access to various wireless technologies such as RF (Radio Frequency), Bluetooth, Wi-Fi, and Zigbee. This capability can allow embedded systems using the 8051 to communicate with other devices or remote servers and send or receive data in real-time.
Interfacing the 8051 microcontroller with wireless communication hardware requires specific communication protocols, depending on the wireless technology used. Additionally, developers need to understand the hardware and software architecture of the wireless device and how its interaction with the 8051 microcontroller can affect the functioning of the whole system.
These advanced topics in embedded C programming for the 8051 microcontroller can increase the capabilities, performance, and safety of embedded systems implemented using the microcontroller. Therefore, developers who master these topics can create reliable, secure, and high-performing embedded systems for a variety of applications.
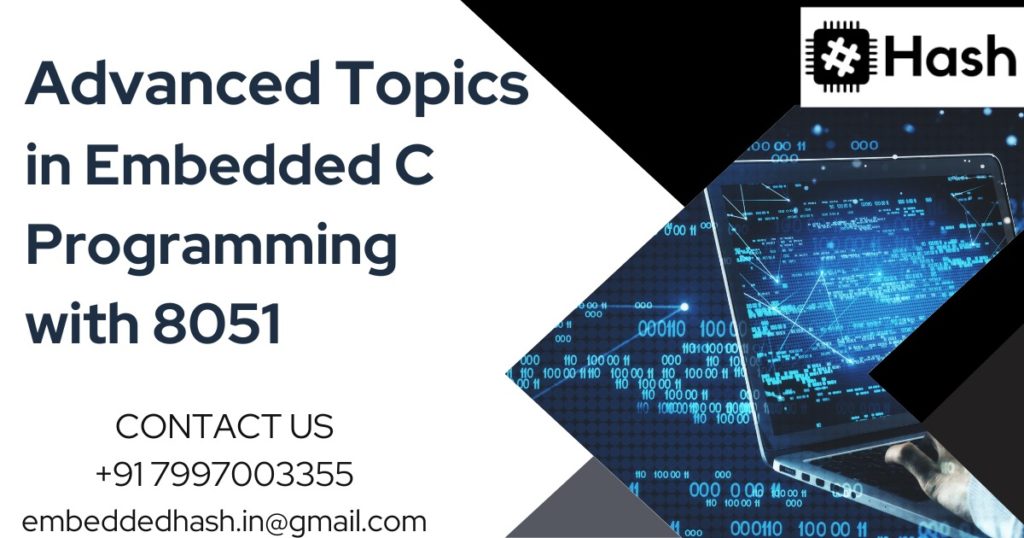
Memory Organization in the 8051 Microcontroller
Memory organization in the 8051 microcontroller is important to understand when it comes to managing and optimizing memory usage in embedded C programming with 8051. The 8051 microcontroller has a segmented memory model, divided into several memory spaces:
- Program Memory: Stores the program instructions that the CPU fetches and executes. In the 8051, the program memory is divided into multiple banks, each containing a fixed number of 8-bit instructions.
- Data Memory: Used to store data and variables during program execution. The data memory is further divided into three regions: Internal RAM, Special Function Register (SFR) space, and bit-addressable memory.
- External Data Memory: The 8051 microcontroller can also access external data memory through address lines and control signals. This external memory is often used for storing large amounts of data or additional program code.
Understanding the memory organization in the 8051 microcontroller allows developers to allocate and utilize memory resources efficiently.
Memory Access and Addressing Modes
Memory access and addressing modes play a crucial role in embedded C programming with 8051 microcontrollers. The 8051 microcontroller provides various addressing modes to access different types of memory.
- Direct Addressing: The simplest form of addressing, where the memory location is specified directly in the instruction.
- Register Indirect Addressing: In this mode, a register is used to hold the memory address, and the instruction operates on the data at that address.
- Indexed Addressing: Allows the use of an index register, such as the DPTR or a register pair, to indirectly access memory locations.
- Immediate Addressing: Data is included as part of the instruction itself without specifying a memory location.
- Bit Addressing: The 8051 microcontroller provides bit-addressable memory, allowing individual bits to be accessed and modified independently.
Developers need to understand and utilize the different addressing modes as per their specific requirements to optimize memory access and speed up program execution.
Optimizing Embedded C Code for Performance and Size
Optimizing embedded C code for performance and size is crucial in resource-constrained environments such as the 8051 microcontroller. Here are some techniques to consider:
- Use appropriate data types: Choose the smallest data type that can hold the required range of values to minimize memory usage.
- Minimize the use of global variables: Excessive use of global variables can consume precious memory. Instead, prefer local variables.
- Use bitwise operations: Bitwise operations can be more efficient when dealing with individual bits or flags rather than using boolean variables or multiple boolean operations.
- Avoid unnecessary conversions: Minimize unnecessary data type conversions, as they can introduce overhead and reduce performance.
- Optimize loops: Optimize loops by reducing iterations, minimizing loop overhead, and avoiding unnecessary calculations within loops.
- Use inline functions: Inline functions can eliminate the overhead of function calls and improve performance in some cases.
- Enable optimization flags: Enable compiler optimization flags to let the compiler apply various optimizations, such as loop unrolling, constant folding, or code size optimization.
- Profile and measure: Profile your code and measure its performance to identify potential bottlenecks and areas for optimization.
Dealing with Limited Resources
The 8051 microcontroller has limited resources, such as memory, I/O ports, and processing power. Here are some strategies for dealing with these limitations and optimizing resource usage:
- Optimize memory usage: Use data types and memory allocation techniques that minimize memory usage. Avoid unnecessary duplication of code or data.
- Prioritize critical functions: Identify critical functions and optimize them for performance. These functions usually include time-critical operations or frequently used functionalities.
- Use interrupts: Utilize interrupts to handle time-sensitive tasks or external events, allowing the CPU to continue with other operations.
- Use timers and counters efficiently: Take advantage of the timers and counters available in the 8051 microcontroller to offload timekeeping and event-counting tasks from the CPU.
- Optimize I/O operations: Minimize I/O operations and use efficient I/O handling techniques, such as buffering, to reduce the overhead of data transfer.
- Use code optimization techniques: Employ optimization techniques discussed earlier, such as minimizing unnecessary calculations, reducing loop iterations, and optimizing data structures.
By being mindful of limited resources and employing optimization techniques, developers can effectively utilize the capabilities of the 8051 microcontroller and develop efficient and reliable embedded C code.
Conclusion
In conclusion, this comprehensive guide has provided a deep understanding of embedded C programming with 8051 microcontrollers, enabling you to build robust systems with confidence. By mastering the concepts, techniques, and best practices outlined in this article, you are well-equipped to embark on exciting projects and create innovative embedded systems.
Throughout this guide, we explored the fundamentals of embedded C programming, from understanding the 8051 architecture to interfacing peripherals and optimizing memory usage. We delved into real-world applications, allowing you to apply your newfound knowledge to practical projects.
As technology continues to advance, embedded systems powered by the 8051 microcontroller will remain integral to numerous industries. By continually expanding your skills and staying up-to-date with emerging trends and advancements, you can remain at the forefront of this dynamic field.
FAQs on embedded C programming with 8051 microcontroller
Embedded C programming is a specialized form of programming used to develop software for embedded systems. It is important for building robust systems with the 8051 microcontroller as it allows developers to control and utilize the microcontroller’s features effectively, ensuring efficient resource management, real-time performance, and reliable operation.
While the 8051 microcontroller can be programmed using standard C language, there are certain embedded C extensions and specific guidelines that should be followed for optimizing code size, memory usage, and timing constraints. There are also specialized development tools and compilers available for embedded C programming with the 8051 microcontroller.
Choosing the right development tools involves considering factors such as the features provided, compiler efficiency, debugging capabilities, simulator support, and compatibility with the 8051 microcontroller. It is recommended to select tools that offer a comprehensive integrated development environment (IDE) with good technical support and documentation.
Designing hardware interfaces requires considering factors such as voltage levels, signal compatibility, noise suppression, power supply requirements, and proper grounding. It is crucial to develop schematics and PCB layouts that adhere to best practices and ensure reliable communication between the microcontroller and external devices or sensors.
Memory optimization techniques include reducing variable sizes, using efficient data types, applying code and data banking techniques, storing constants in ROM, and utilizing linker optimizations. Additionally, disabling unnecessary compiler optimization options, such as floating-point support, can save valuable memory resources.
Best practices for debugging embedded C programs on the 8051 microcontroller include using in-circuit emulators, hardware breakpoints, real-time debugging tools, and software debugging techniques like logging and serial communication. It is also helpful to analyze program flow, monitor variables, and use assert statements to catch errors during development.
Ensuring real-time performance involves careful task scheduling, utilizing hardware interrupts, reducing interrupt latency, optimizing code execution time, and managing system resources efficiently. Using techniques such as priority-based scheduling and interrupt-based event handling can help achieve real-time responsiveness.
Testing reliability involves unit testing of individual modules, integration testing of the overall system, stress testing, and verification against specifications. Test cases should cover both normal and exceptional scenarios, with attention to input validation, error handling, and boundary conditions.
To handle power management, it is important to design software that maximizes sleep modes, reduces unnecessary processing, optimizes timers, and minimizes power-hungry peripherals. Techniques like clock gating and power gating can help preserve energy by disabling unused components.
Yes, guidelines include writing modular and reusable code, following a consistent coding style, adding comments and documentation, using common coding guidelines like MISRA C, and leveraging software version control systems for managing code changes and collaboration.